- Documentation
- Video Calling
- Solution Implementation
- iOS
Solution Implementation Guide for iOS
One of the typical uses of a real-time video scene is that multiple users in the same session make a real-time video call. That is, multiple people push and pull each other together, for example:
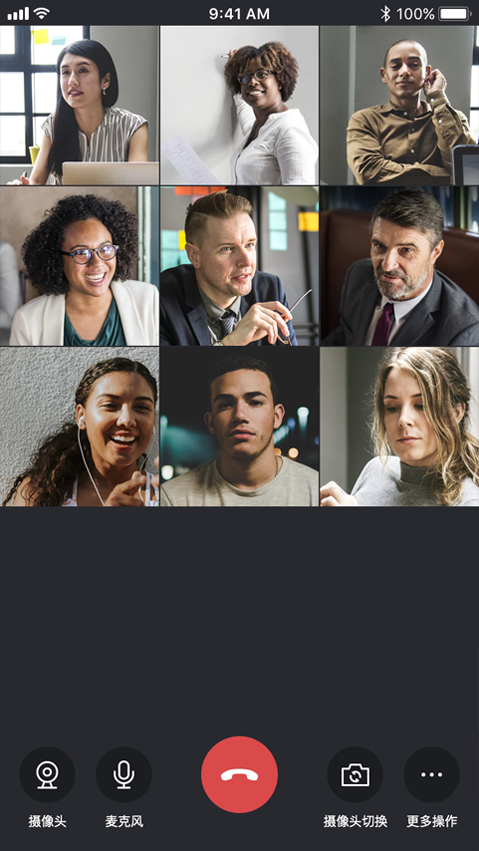)
Taking the scene of a real-time video call between 2 people as an example, the detailed API call sequence diagram of Express Video SDK is as follows:
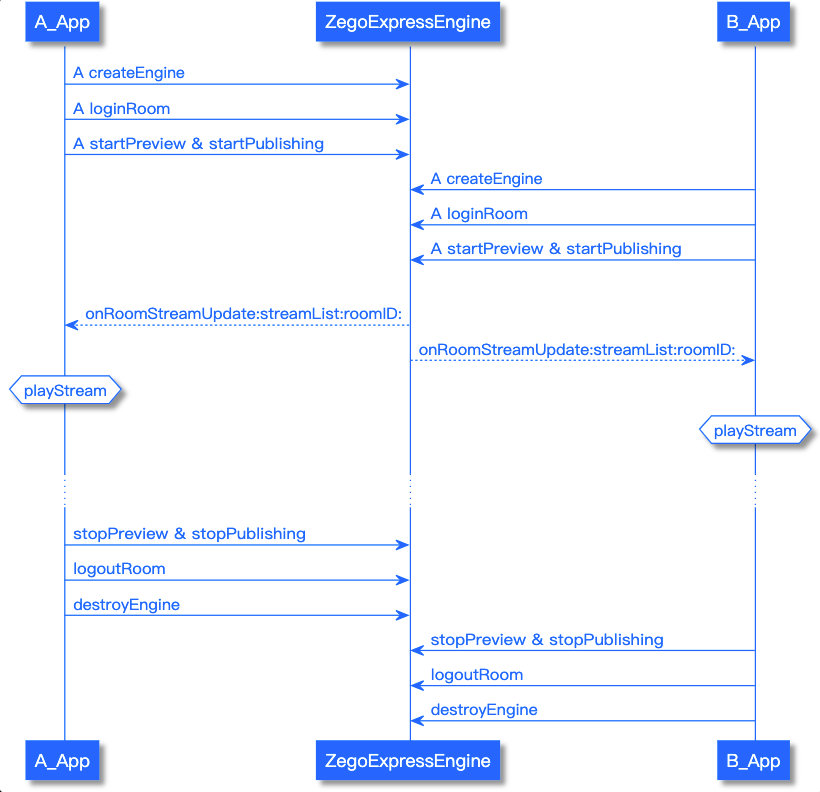)
Please note:
In the above process, the real-time video between 2 room members is taken as an example. In fact, Express Video SDK supports multi-person real-time video. It is recommended that developers refer to the above process to design their own multi-person real-time video call scenes.
In order to make it easier for developers to understand the logic in the
Video Call
module inSample Topics
, the following sections will pick out and explain the functional core source code fragments. Developers can also directly refer to Demo.
1 Initialize The SDK
Before using Express Video SDK to make a video call, you need to initialize Express Video SDK. Since there are many operations handled internally by the SDK, it is recommended that developers do it when the App starts.
The source code snippets related to the initialization in the video call
module are demonstrated as follows, the following sample code is not completely consistent with the source code in the video call
module, for reference only:
// Import the ZegoExpressEngine.h header file
#import <ZegoExpressEngine/ZegoExpressEngine.h>
// Follow the ZegoEventHandler protocol to handle event callbacks you care about
@interface ViewController () <ZegoEventHandler>
// ······
@end
// Fill in appID and appSign
unsigned int appID =; // Please register and get it through the official website, the format is: 1234567890
NSString *appSign =; //Please register and get it through the official website, the format is: @"0123456789012345678901234567890123456789012345678901234567890123" (64 characters in total)
// Create an engine, use a test environment, access to common scenarios, and register self as the eventHandler callback agent
// If you don't need to register the callback, you can pass nil as the eventHandler parameter, and then you can call the "-setEventHandler:" method to set the callback proxy
[ZegoExpressEngine createEngineWithAppID:appID appSign:appSign isTestEnv:YES scenario:ZegoScenarioGeneral eventHandler:self];
2 Log In To The Room And Push And Pull The Stream, Monitor Various Callbacks, And Start A Video Call
Before real-time video conversations between users, they need to log in to the same room. After receiving the callback of successfully logging in to the room, they can directly call the API interface of Express Video SDK for push and pull streaming operations.
A user who has already made a video call in the room needs to obtain a notification of a user who later enters the room for a video call to push the stream, and also needs to obtain a notification of a user who exits the video call halfway to stop the push. After obtaining these notifications, users who have previously made video calls in the room should pull the newly added stream in the room, stop pulling the stopped stream in the room, and display the corresponding UI.
The source code snippets related to the initialization in the video call
module are demonstrated as follows, the following sample code is not completely consistent with the source code in the video call
module, for reference only:
// Create user object
ZegoUser *user = [ZegoUser userWithUserID:@"user1"];
// Start logging in to the room
[[ZegoExpressEngine sharedEngine] loginRoom:@"room1" user:user];
// The following are commonly used room related callbacks
-(void)onRoomStateUpdate:(ZegoRoomState)state errorCode:(int)errorCode extendedData:(NSDictionary *)extendedData roomID:(NSString *)roomID {
// Room status update callback. After logging in to the room, when the room connection status changes (such as room disconnection, login authentication failure, etc.), the SDK will notify you through this callback
}
-(void)onRoomUserUpdate:(ZegoUpdateType)updateType userList:(NSArray<ZegoUser *> *)userList roomID:(NSString *)roomID {
// The user status is updated. After logging in to the room, when a user is added or deleted in the room, the SDK will notify you through this callback
}
-(void)onRoomStreamUpdate:(ZegoUpdateType)updateType streamList:(NSArray<ZegoStream *> *)streamList roomID:(NSString *)roomID {
// The stream status is updated. After logging in to the room, when a user pushes or deletes an audio and video stream in the room, the SDK will notify you through this callback
NSArray<NSString *> *allStreamIDList = [_allUserViewObjectList valueForKeyPath:@"streamID"];
if (updateType == ZegoUpdateTypeAdd) {
for (ZegoStream *stream in streamList) {
ZGLogInfo(@" ???? ???? --- [Add] StreamID: %@, UserID: %@", stream.streamID, stream.user.userID);
if (![allStreamIDList containsObject:stream.streamID]) {
[self addRemoteViewObjectIfNeedWithStreamID:stream.streamID];
}
}
} else if (updateType == ZegoUpdateTypeDelete) {
for (ZegoStream *stream in streamList) {
ZGLogInfo(@" ???? ???? --- [Delete] StreamID: %@, UserID: %@", stream.streamID, stream.user.userID);
[self removeViewObjectWithStreamID:stream.streamID];
}
}
}
In the onRoomStreamUpdate callback, we can get the stream addition/removal information, pull the stream to play startPlayingStream
when the stream is added, and stop pulling the stream when the stream is removed stopPlayingStream
Please refer to the room login process: [Quick Start - Implementation - 2.2
For the streaming process, please refer to: Quick Start - Implementation - 2.3
Note:
- The ʻonPublisherStateUpdate` callback can monitor the push stream status. If the push is unsuccessful, it is generally a network problem, and the SDK will do retry work. Developers can also make a limited number of push retry according to the situation, or give a friendly interactive prompt.
Please refer to the pull flow process: Quick Start - Implementation - 2.4
Note:
onPlayerStateUpdate
can monitor the streaming state. If the streaming is unsuccessful, it is generally a network problem, and the SDK will do retry work. The developer can also do a limited number of streaming retries according to the situation, or give a friendly interactive prompt.
3 Stop Video Call
When the user exits the video call during the video call, he should stop streaming, stop streaming, exit the room, and release the corresponding UI object resources.
The relevant source code snippets in the video call
module are demonstrated as follows, the following sample code is not completely consistent with the source code in the video call
module, for reference only:
// stop streaming
[[ZegoExpressEngine sharedEngine] stopPublishingStream];
// stop local preview
[[ZegoExpressEngine sharedEngine] stopPreview];
//Stop streaming, if you pull multiple streams, you should traverse and stop all streaming
engine.stopPlayingStream(streamID);
// Set the corresponding pull-stream rendering View to null, release resources, etc.
...
/** Log out of the room */
engine.logoutRoom("room1");